DATA COLLECTION
There are four built-in collections of data types in Python, each one with different qualities for particular purposes.
LISTS:
– Lists are used to store multiple items in a single variable and are created using square brackets [ ].
– List items can be ordered, they are changeable, allow duplicate values, and can contain any data type.
– List items are indexed, the first item has index [0], the second item has index [1], and so on.
– ” : ” colon is used inside the index as a range.
– To check the type of list we are using we write print (type(list_name))
Example #1:
numbers = [“1”, “2”, “3”]
print(numbers[0])
Output:
>> 1
Example #2:
list1 = [“letter”, 1.4, True, False, “name”, 17]
print(list1[1:4])
Output:
>> [1.4, True, False]
TUPLES:
Tuples are used to store multiple items in a single variable, however, they are a collection that is ordered and unchangeable.
As opposed to the list syntax, the tuples are written with round brackets ( ).
They can contain multiple data types as well.
Example #1:
numbers = (“1”, “2”, “3”)
print(numbers[2])
Output:
>> 3
Example #2:
numbers = (“1”, “2”, “3”)
print(type(numbers))
>> <class ‘tuple’>
SETS:
A set is a collection of data types that is unordered, unchangeable, unindexed, and duplication is not allowed.
A set uses curly brackets { }.
Example #1:
numbers = {“1”, “2”, “3”}
print(numbers[2])
Output:
>> TypeError: ‘set’ object is not subscriptable #Error due to unindexation.
Example #2:
numbers = {“1”, “2”, “3”}
print(numbers)
Output:
>> {‘3’, ‘2’, ‘1’}
DICTIONARIES:
They are ordered, changeable, and do not accept duplicates.
Dictionaries are used to store data values in key : value pairs, and are written with curly brackets.
A visual example of key: value pairs:
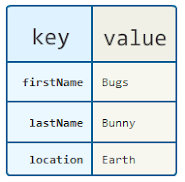
Example #1:
variable.append (adds a new object)
variable.insert (index, object)
variable.remove (specific object)
variable.index(object in list) #returns index number.
variable.count (object)
variable.sort ( )
variable.reverse( )
variable.copy ( )
Mapping method for Dictionaries: variable.get (key) # Returns value pair