Brief Definition:
Python apart from being the name of a snake type, it’s an interpreted, object-oriented, high-level programming language with dynamic semantics. It’s widely used for data structures, machine learning, artificial intelligence, database management, app development, scripting, web development, etc. It’s an outstanding programming language because its syntax emphasizes readability to make it more understandable for the users.
DATA TYPES:
In order for the Python interpreter to understand numbers or letters we use the “Data Types” which are a classification of built-in words that can store mathematical numeric values and strings, each one is different and has its logical purpose.
Below are listed the most used ones:
- Strings: Holds letters (str) | x = “Hello world”
- Integers: Holds whole numbers (int) | x= 1
- Float: Holds short decimals (float) | x = 1.5
- Boolean: Holds either True or False statements (bool) I x = True (or False)
Casting: Used to temporarily assign different datatypes.
Note: The pipe sign | represents an alternative.
x = int(1.5) | x= float( 1) |x = str(1)
print(x)
The output will be = 1 | 1.0 | “1”
String Modification: Are called methods as opposed to functions, they seem the same but
always belong to a string or any other argument.
x= “Hi There”
print(x.upper()) | print(x.lower())
Output= HI THERE | hi there
There are more alternatives to modify a string, it depends on what you want to do with it.
ARITHMETIC OPERATORS:
Note: ” | ” represents separation.
+ | Addition | x + y
– | Subtraction | x – y
* | Multiplication | x * y
/ | Division | x / y
% | Modulus | x % y
** | Exponentiation | x ** y
// | Floor division | x // y
IF STATEMENTS & CONDITIONALS:
Equals | a == b
Not Equals | a != b
Less than | a < b
Greater than | a > b
Less than or equal to | a <= b
Greater than or equal to | a >= b
And | a and b
Or | a or b
Example #1:
Therefore this example above will print “x & y are equal”.
Example #2:
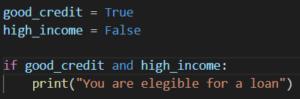
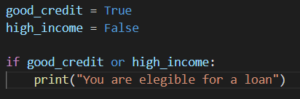